In the world of smart contract development, there are certain security checks and validations that are impossible to implement in on-chain EVM execution. These limitations often leave protocols vulnerable to sophisticated attacks. However, with the Credible Layer, we can now implement these previously impossible assertions.
The limitations mentioned above exist because Solidity is designed to work within the constraints of the Ethereum Virtual Machine (EVM), which was built for simple, deterministic execution. The EVM provides a limited set of operations and doesn't expose internal transaction details like the call stack or intermediate state changes. This design choice was made to ensure predictable gas costs and execution behavior, but it means that certain security checks that would be trivial in traditional software development become impossible in on-chain EVM execution.
Understanding The Credible Layer
Before diving into the examples, it's important to understand that the Credible Layer provides powerful cheatcodes (native functions) that extend the EVM's capabilities. These cheatcodes enable:
- State Management: Accessing pre and post transaction states
- Transaction Data: Inspecting call inputs and logs
- Storage Operations: Reading and tracking storage changes
- Trigger System: Executing assertions based on specific events
What makes these capabilities particularly powerful is that they can be used to implement security checks that are completely independent of the protocol's code. This means that even if there are bugs or vulnerabilities in the original contracts, the assertions will still enforce the intended security guarantees. Simplified, this works by telling the blockbuilder, which contract a specific assertion is enforced on, but having the assertions stored separate from the smart contract itself.
Additionally, assertions can be added after deployment without modifying the original contracts, providing a simple and powerful way to enhance security without complex upgrade mechanisms.
1. Intra-Transaction Oracle Price Deviation Detection
One of the most common attack vectors in DeFi is oracle price manipulation within a single transaction. In on-chain EVM execution, it's impossible to track and validate price changes that occur during the execution of a transaction. However, with the Credible Layer, we can implement assertions that detect suspicious price deviations and other state changes in the call stack of each transaction.
This code example shows how to prevent oracle price manipulation by checking that all price updates within a transaction stay within a defined deviation range (e.g., ±10%) from the initial price.
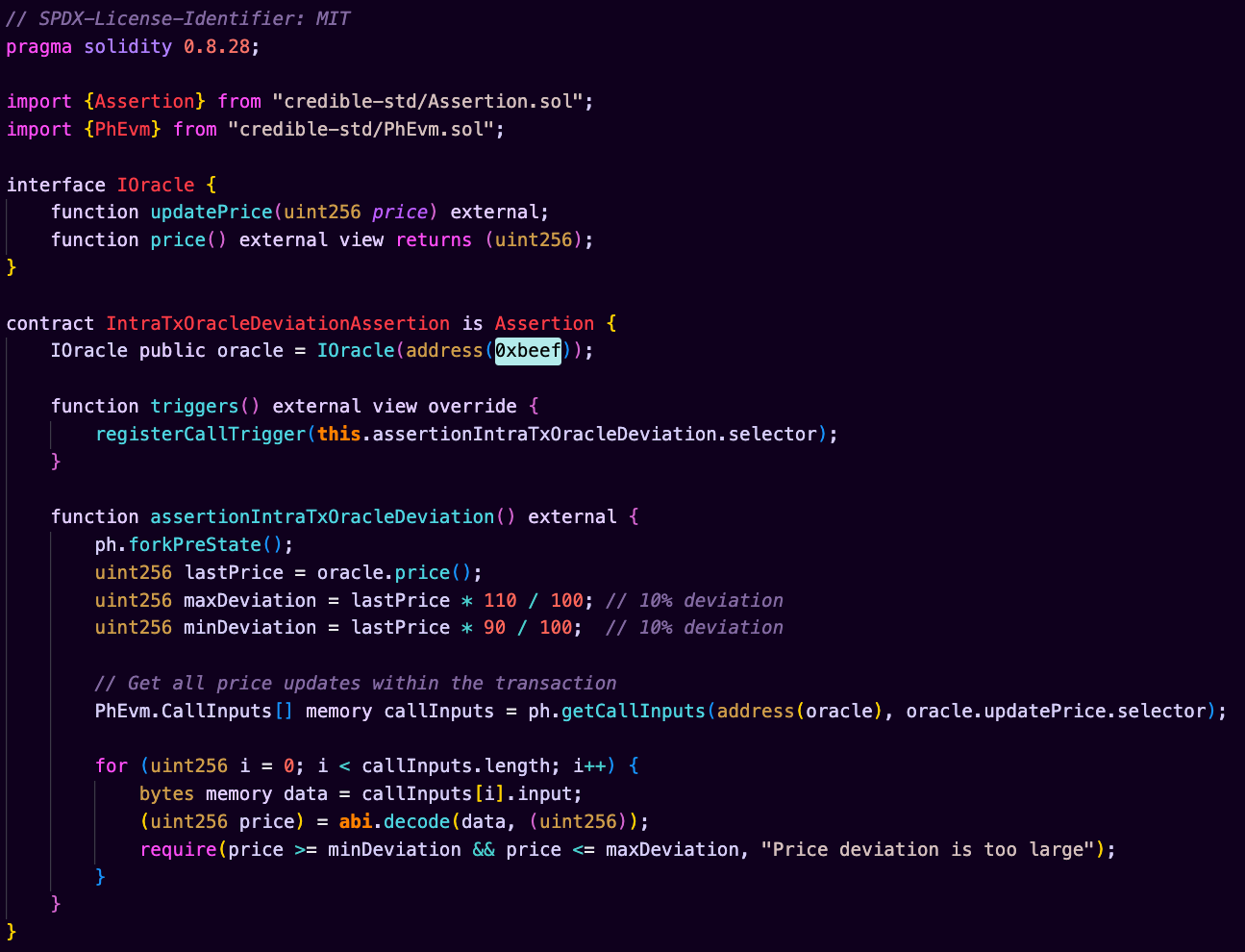
This assertion is impossible in on-chain EVM execution because:
- Solidity cannot track all function calls within a transaction
- The EVM doesn't provide a way to inspect the call stack during execution
Implementation Notes:
- Uses
getCallInputs()
to capture all oracle price updates within the transaction - Leverages
forkPreState()
to establish a baseline price - Provides real-time monitoring of price changes during transaction execution
2. Storage Slot Manipulation Prevention
In proxy contracts and upgradeable contracts, storage slot manipulation through delegate calls is a significant risk. On-chain EVM execution cannot prevent unauthorized changes to storage slots, but the Credible Layer can track and validate all storage changes.
This code example shows how to prevent unauthorized changes to a proxy contract's implementation slot by monitoring all storage changes and reverting if any modifications are detected.
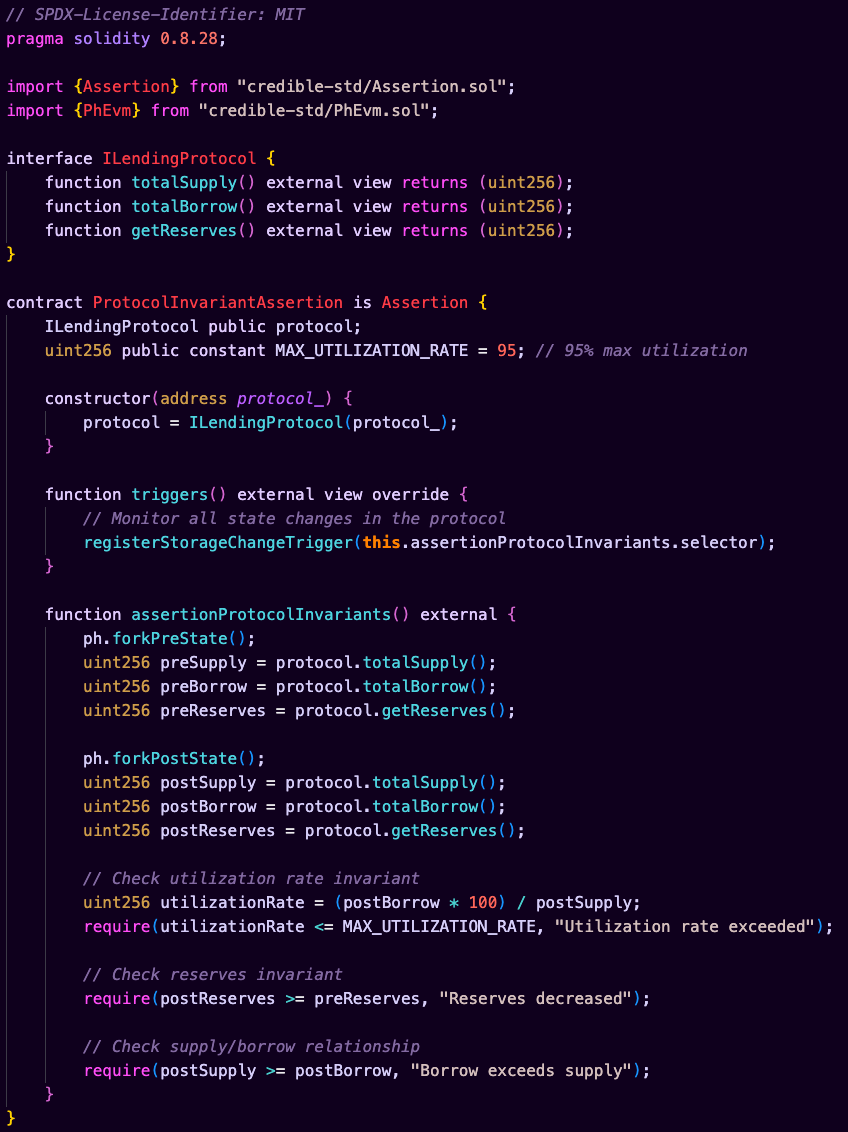
This assertion is impossible in on-chain EVM execution because:
- Solidity cannot track all storage changes within a transaction
- There's no way to prevent delegate calls from modifying storage slots
- The EVM doesn't provide a way to monitor storage changes during execution
Implementation Notes:
- Uses
registerStorageChangeTrigger()
to monitor specific storage slots - Leverages
getStateChangesAddress()
to track all changes to the implementation slot - Provides granular control over which storage slots to monitor
- Can be used to protect critical storage slots from unauthorized modifications
3. Call Stack Owner Address Validation
In complex protocols, ensuring that certain operations can only be performed by specific addresses in the call stack is crucial. On-chain EVM execution can only check the immediate caller (msg.sender
), but the Credible Layer can inspect the entire call stack.
This code example shows how to prevent unauthorized access by checking every address in the call stack and reverting if a restricted address is found at any level.
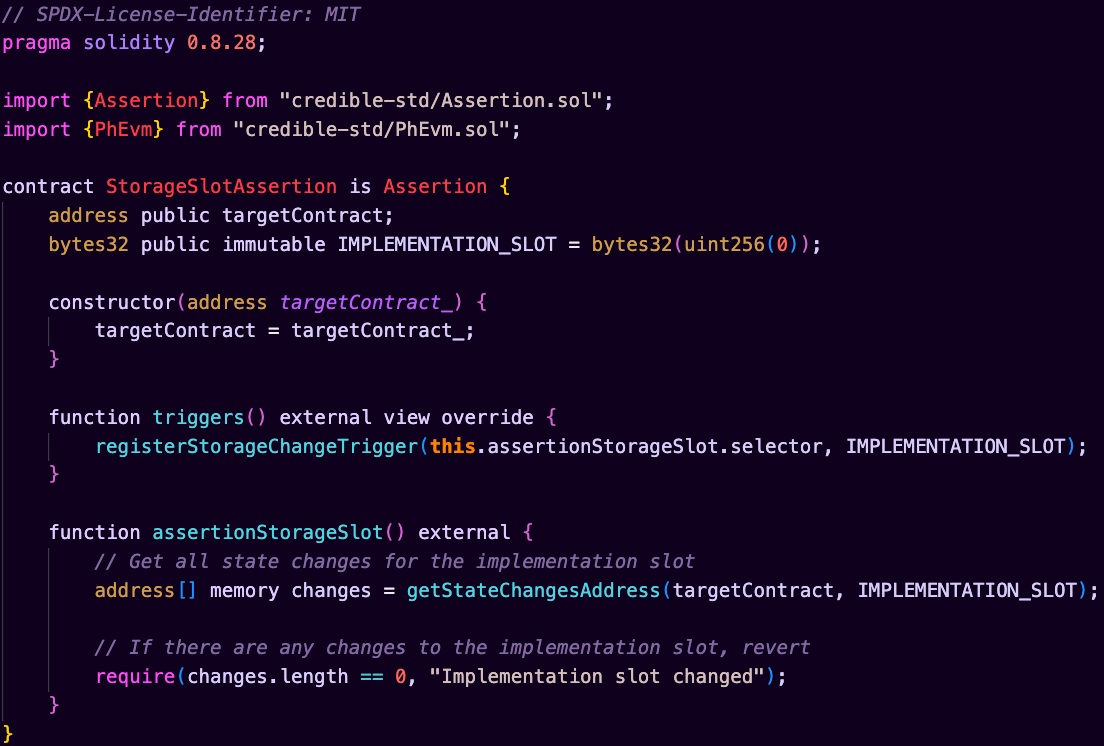
This assertion is impossible in on-chain EVM execution because:
- Solidity can only access
msg.sender
(immediate caller) - There's no way to inspect the call stack during execution
- The EVM doesn't provide a way to track the call hierarchy
Implementation Notes:
- Uses
getCallInputs()
to capture all calls to the protocol - Provides access to the complete call stack through
CallInputs
struct - Can validate caller addresses at any level in the call hierarchy
- Useful for preventing unauthorized access through complex call paths
4. Post-Deployment Protocol Invariant Enforcement
One of the most powerful aspects of the Credible Layer is the ability to enforce protocol-wide invariants even after contract deployment. This is particularly valuable when you need to add safety checks to existing protocols or when you want to enforce invariants across multiple contracts without modifying the original code.
This code example shows how to enforce critical lending protocol invariants by checking utilization rates, reserve requirements, and supply/borrow relationships across state changes.

This example demonstrates several key advantages of using assertions for protocol invariants:
- Post-Deployment Safety: The assertion can be added to an existing protocol without modifying the original contracts. This is particularly useful for:
- Adding safety checks to protocols that are already deployed
- Enforcing invariants across multiple contracts without complex upgrade mechanisms
- Implementing emergency circuit breakers without contract modifications
- Cross-Contract Validation: The assertion can monitor state changes across multiple contracts and enforce relationships between them, which would be difficult to implement in the original contracts without complex cross-contract calls.
- Flexible Monitoring: The assertion can be updated or replaced without requiring protocol upgrades, making it easier to adapt to new security requirements or discovered vulnerabilities.
- Independent Verification: The assertion runs independently of the protocol's logic, providing an additional layer of security that can't be bypassed by bugs in the original contracts.
Implementation Notes:
- Uses
registerStorageChangeTrigger()
to monitor all state changes - Leverages
forkPreState()
andforkPostState()
for state comparison - Can enforce complex relationships between multiple state variables
- Provides a way to add safety checks without modifying existing contracts
Conclusion
These four examples demonstrate the power of the Credible Layer in implementing security checks that were previously impossible in on-chain EVM execution. By providing access to transaction state, call stack information, storage changes, and the ability to enforce protocol-wide invariants, the credible layer enables developers to implement more robust security measures and prevent sophisticated attacks.
What makes these assertions particularly powerful is their ability to:
- Enforce security guarantees that are impossible to implement in on-chain EVM execution
- Add security checks after deployment without modifying the original contracts
- Monitor and enforce invariants across multiple contracts in a way that would be vulnerable to race conditions or other attacks if implemented in the contracts themselves
- Provide an independent layer of security that cannot be bypassed even if there are bugs in the original contracts
The ability to implement these assertions is particularly valuable for:
- DeFi protocols that need to protect against price manipulation
- Upgradeable contracts that need to prevent unauthorized implementation changes
- Complex protocols that require strict access control across the entire call stack
- Existing protocols that need additional safety checks without contract modifications
As the blockchain ecosystem continues to evolve, tools like the Credible Layer will play an increasingly important role in securing smart contracts and protecting user assets.